At this post I will tell you how to create your own Image Gallery or Photo Gallery at your desktop.
Explanation (why?).First of all I wanted to have dinamically changed images on my desktop. But it was only my dream. Once upon a time I downloaded from the Internet about 500 art works by some well known artists :) Then I understood that I want to see it one by one (for example 1 per day) at my desktop. That is why I created this solution.
How it works?I have created Html page with black backround and with just one image. This image fit browser window. All gallery images are called the same way: ***.jpg where *** - is a number with leading zeroes. These images are placed into "Images" folder, so Html page can load them by the number. All manipulations are organized using JavaScript. I added small setup window for this page, which you can see just clicking at + button at the top left corner of this page. And, at the end, the state of the page I save using Cookies.
And:
1. Active Desktop in Windows allows us to set html page as desktop image.
2. This html page was developed for IE8 browser, so, at other browsers it may fails. But we actually need just IE.
How to use:
1. Copy the following Html code to some html file. (To do it open notepad and save the following as file named index.html)
<html>
<head>
<script>
// global variables
var screenBorder = 50;
var screenX;
var screenY;
var $get;
var minImage = 1;
var maxImage = 5;
var imageIndex = 1;
var loadMode = "Random";
var image = new Image();
function createCookie(name,value,days) {
if (days) {
var date = new Date();
date.setTime(date.getTime()+(days*24*60*60*1000));
var expires = "; expires="+date.toGMTString();
}
else var expires = "";
document.cookie = name+"="+value+expires+"; path=/";
}
function readCookie(name) {
var nameEQ = name + "=";
var ca = document.cookie.split(';');
for(var i=0;i < ca.length;i++) {
var c = ca[i];
while (c.charAt(0)==' ') c = c.substring(1,c.length);
if (c.indexOf(nameEQ) == 0) return c.substring(nameEQ.length,c.length);
}
return null;
}
function eraseCookie(name) {
createCookie(name,"",-1);
}
// save state to cookies
function saveState()
{
createCookie("minImage", minImage, 3650);
createCookie("maxImage", maxImage, 3650);
createCookie("imageIndex", imageIndex, 3650);
createCookie("loadMode", loadMode, 3650);
}
// load state from cookies
function loadState()
{
var tmp = readCookie("minImage");
if (tmp) minImage = parseInt(tmp);
tmp = readCookie("maxImage");
if (tmp) maxImage = parseInt(tmp);
tmp = readCookie("imageIndex");
if (tmp) imageIndex = parseInt(tmp);
tmp = readCookie("loadMode");
if (tmp) loadMode = tmp;
}
function showSetupWindow()
{
loadState();
$get('firstImg').value = minImage;
$get('lastImg').value = maxImage;
if (loadMode == "Random")
$get('randomButton').checked = true;
else
$get('currentButton').checked = true;
$get("setupWindow").style.visibility = "visible";
}
function closeSetupWindow()
{
// TODO: save state from window to variables.
//
minImage = parseInt($get('firstImg').value);
maxImage = parseInt($get('lastImg').value);
if ($get('randomButton').checked === true)
loadMode = "Random";
else
loadMode = "Current";
saveState();
$get("setupWindow").style.visibility = "hidden";
}
function prev()
{
imageIndex--;
if (imageIndex < minImage)
imageIndex = maxImage;
loadImage(imageIndex);
}
function next()
{
imageIndex++;
if (imageIndex > maxImage)
imageIndex = minImage;
loadImage(imageIndex);
}
function bodyLoad()
{
$get = document.getElementById;
screenX = document.body.clientWidth;
screenY = document.body.clientHeight;
loadState();
if (loadMode == "Random")
imageIndex = Math.round(Math.random()*(maxImage-minImage))+minImage;
saveState();
loadImage(imageIndex);
}
function loadImage(imageIndex)
{
var screenX = document.body.clientWidth - 60;
var screenY = document.body.clientHeight - 40;
var num = imageIndex;
if (imageIndex < 10) num = "0" + num;
if (imageIndex < 100) num = "0" + num;
image.onload = function(){
image.style.position = "absolute";
var imageWidth = image.width;
var imageHeight = image.height;
var img = document.getElementById('image1');
img.onload = function()
{
if ((screenX/imageWidth)*imageHeight <= screenY)
{
img.width = screenX;
img.height = (screenX/imageWidth)*imageHeight;
img.style.top = Math.round((screenY - (screenX/imageWidth)*imageHeight)/2 + 20) + "px";
img.style.left = "30px";
}
else
{
img.width = (screenY/imageHeight)*imageWidth;
img.height = screenY;
img.style.top = "40px";
img.style.left = Math.round((screenX - (screenY/imageHeight)*imageWidth) / 2 + 30) + "px";
}
}
img.src = image.src;
}
var desc = document.getElementById('desc1');
image.src = "images/" + num + ".jpg";
}
</script>
</head>
<body bgcolor="#000000" onload="bodyLoad();">
<img id="image1" style="position: absolute; top: 100px; left: 100px;" />
<a style="position: absolute; top: 10px; right: 10px; color: White;" href="http://serpblog.blogspot.com/2008/03/active-desktop-or-image-gallery-for.html" target="_blank">Home (serp)</a>
<input type="button" value="+" onclick="showSetupWindow();" />
<div id="setupWindow" style="visibility: hidden; padding: 10px; overflow: hidden; position: absolute; top: 0px; left: 0px; width: 295px; height: 170px; background: black; border: 2px solid green; color: #FFFFFF;">
<div style="position: absolute; top: 10px; left: 10px;">First image:</div>
<input id="firstImg" style="position: absolute; top: 10px; left: 100px;" id="firstImage" size="5" style="float: right;" type="text" />
<div style="position: absolute; top: 30px; left: 10px;">Last image:</div>
<input id="lastImg" style="position: absolute; top: 30px; left: 100px;" id="lastImage" size="5" type="text" />
<input style="position: absolute; top: 60px; left: 10px; width: 150px;" type="button" name="prev" onclick="prev();" value="Previous image" />
<input style="position: absolute; top: 90px; left: 10px; width: 150px;" type="button" name="next" onclick="next();"value="Next image" />
<div style="position: absolute; top: 10px; left: 190px;">Load mode:</div>
<div style="position: absolute; top: 40px; left: 210px;">Random</div>
<input id="randomButton" style="position: absolute; top: 40px; left: 180px;" type="radio" name="loadMode" value="Random"/>
<div style="position: absolute; top: 60px; left: 210px;">Current</div>
<input id="currentButton" style="position: absolute; top: 60px; left: 180px;" type="radio" name="loadMode" value="Current" />
<input style="position: absolute; top: 130px; left: 10px; width: 270px;" type="button" onclick="closeSetupWindow();" value="Save and close" />
</div>
</body>
</html>
2. At the folder where you saved
index.html create
Images folder and create there five files 001.jpg 002.jpg 003.jpg 004.jpg and 005.jpg
To do it I prepared some images for you:
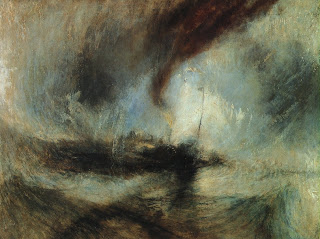
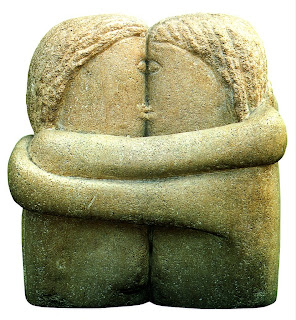
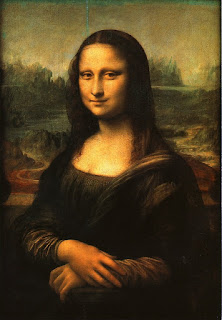
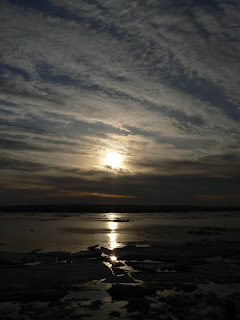
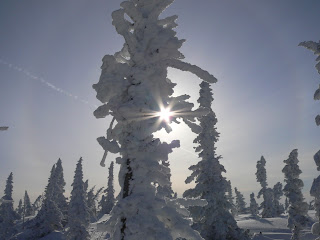
3. Right click on your desktop, choose options - desktop and change your current image to index.html page, you have created.
4. Have fun :)
What else?
Using setup window (just clicking on + button at top left corner of your desktop) you may change amount of images into your collection increasing Last Image property.
Also you may divide your collection to some parts and load one of them using First Image and Last Image properties.
You may navigate forward and backward into current collection of images using "Next image" and "Previous image" buttons.
You may freeze current displayed image using "Current" radio button.
Future.
I see the future... :) A lot of options like - background color.
For every image we aslo shows description file. This may be interesting for some art works.
Or may be new site with a number of large collections of desktop images. So user just chooses collection, but all images are stored on the internet, and user just download one by one, for example one per day =)
Or something else, who knows?....
P.S. If you have some problems or ideas please fell free to describe it here.